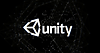
Lesson 3 - Coding in C# (General)
C# is a really important language to learn if you want to get into computer science. It is a really popular programming language to create application and games (most popular being Unity). Won’t be going too in depth in every topic. If you would like to learn more I'd recommend you go to W3school and check out their course.
C# Output
When programming the first thing you learn is how to print “Hello World” in your output. If you did Roblox scripting before you know that Lua uses print() to print out to the output. But for C# we use WriteLine().
WriteLine Syntax:
Console.WriteLine("Hello World"); //prints out Hello World in output
When you run this in your complier you will notice that it will create a new line once your output. If you don’t want a new line to be create just remove the Line in WriteLine();
Write Syntax:
Console.Write("Hello World"); //prints out Hello World in output without creating a new line
C# Variables
A variable in any programming language is a container stores the value of something else and is able to change.
Example:
Lets say we have camera Position in unity and we have a game object that the player is able to move. We can’t use numbers for the camera position since the game object constantly moves. So we can use a variable to track the position of the game object and set it to our camera.
Syntax:
type variableName = value;
Creating/Declaring a variable
To declare a variable we need to specify what data type we want to use. If you are coming from a language that doesn’t need to specify what data type you want to use like JavaScript, Lua, and Python. Where they use var and local. But for C# and some other programming languages you need to specify what data type you want to use.
Data Types:
-
Integer: int
-
Any whole number such as 72 or -102
-
-
Double/Float: double/float
-
Any decimal such as 3.14 or -0.25
-
-
Char: char
-
A single character like ‘A’ or ‘a’
-
-
String: string
-
An array or list of characters like “Hello”
-
-
Boolean: bool
-
true or false
-
Variable Examples:
int playerCoins = 5;
float playerHP = 125.25;
string playerName = "Captain_Script";
char playerGrade = 'A';
bool notDead = true;
C# User Input
To accept user input in C#, we use the ReadLine Method of Console.
string playerName = Console.ReadLine(); //accepts a string value
Console.WriteLIne("Hello, " + playerName);
But the issue is if you want to accept use input for another data type like int, it will print out this error “Cannot implicitly convert type 'string' to 'int’”. So instead, we need to convert the ReadLine to a int so we used Convert.ToInt32.
int playerAge = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("The player is :" + playerAge);
C# Operators
In C# operators are used to perform operations on variables or values. In this section I’ll be showing user Arithmetic, Assignment, Comparison, and Logical operators.
Arithmetic Operators
Operators Description Example
+ Adds together two values x + y
- Subtracts one value from another x - y
* Multiplies two values x * y
/ Divides two values x / y
% The Remainder of 2 numbers x % y
++ Same as value + 1 x++
-- Same as value - 1 x--
Comparison Operators
Operators Example Same As
= x = 5 x = 5
+= x += 5 x = x + 5
-= x -= 5 x = x - 5
*= x *= 5 x = x * 5
/= x /= 5 x = x / 5
%= x %= 5 x = x % 5
Assignment Operators
Operators Example
== x == 5
!= x != 5
> x > 5
< x < 5
>= x >= 5
<= x <= 5
Logical Operators
Operators Example Description
&& x > 5 && x < 2 Returns true if both conditions are true
|| x > 5 || x < 2 Returns true if both conditions are false
! !x Opposite of the condition
C# If Statements
The If Statement is a statement that checks if the condition is true to run the following code. The Logical Operators that we learned in the previous section are used to check if a condition is true or not
if, else if, else Statement Syntax
if (condition)
{
//print out if condition is true
}
else if (condition2)
{
//print out if condition2 is true and condition is false
}
else
{
//else print this
}
if statement example
int a = 5;
int b = 3;
if (a > b && a % 2 == 0)
{
Console.WriteLine("A is greater then b and is a even number");
}
In this case since a is greater than b but not a even number the print statement won’t run since we use the and operator. When we're using the and operator all the conditions need to be true for the entire thing to be true.
else Statement
The else statement gets fired if the condition is false. S it would only run if our conditions is false, if it is true it won’t run at all.
int a = 5;
int b = 3;
if (a > b && a % 2 == 0)
{
Console.WriteLine("A is greater then b and is a even number");
}
else
{
Console.WriteLine("Our condition is false");
}
else if statement
The else if statement is a statement that gets fired if the first condition is false and the next condition is true.
int a = 5;
int b = 3;
if (a > b && a % 2 == 0)
{
Console.WriteLine("A is greater then b and is a even number");
}
else if(a > b && a % 2 != 0)
{
Console.WriteLine("A is greater then b and is a odd number");
}
else
{
Console.WriteLine("Our condition is false");
}
So, in this case it will print out "A is greater then b and is a odd number” since our else if condition is true and our if statement is false
C# While Loop
A while loop is type of loop that loops until the condition becomes false.
while (condition)
{
//code
}
Example of C# While Loops
int i = 5;
while(i > 0)
{
Console.WriteLine("Yes");
i--;
}
In this example “Yes” will get printed out 5 times.
C# For Loop
The For loop is another type of loop you will see pretty often in coding. Instead of running until the condition becomes false. The for loop runs a certain number of times.
for(statement1; statement2; statement3)
{
//code
}
● statement1: declare a variable
● statement2: the condition
● statement3: increment
Example of C# For loops
for(int i = 0; i < 5; i++)
{
Console.WriteLine(i);
}
Prints out 1 2 3 4 5 on new lines
C# Break and Continue
The break keyword allows you to “break” out of any loop and skips it.
The continue keyword is similar to break but it just skips one literation and “continues” with the loop.
Example 1:
for(int i = 0; i < 5; i++)
{
if(i == 3)
{
break;
}
Console.WriteLine(i);
}
Example 2:
for(int i = 0; i < 5; i++)
{
if(i == 3)
{
continue;
}
Console.WriteLine(i);
}
In example 1, it only prints out 0 1 2 because we leave the loop when i is equal to 3
In Example 2, it prints out 0 1 2 4 because it skips 3.
C# Arrays
An array stores multiple value of the same data type.
string[] players = {"Captain_Script", "NoobMaster123"};
Changing an Array Element
players[1] = "C#IsCool"; //NoobMaster123 gets replaced by C#IsCool
Iterating through an Array
There is a special type of for loop that is specially designed to loop through elements of an array.
foreach(string i in players)
{
Console.WriteLine(i);
}
This will print out Captain_Script and NoobMaster123
C# Methods
A method is blocks of reusable code. You are able to pass parameters and return values to do something in your game.
public static int myMethod(int a, int b)
{
return a + b;
}
int method = myMethod(5,5);
Console.WriteLine(method);
In this method we are passing in two ints a and b and returning the value of a + b. So in this example the code will print out 10.
C# OOP
OOP stands for Object-Oriented Programming, which provides a clearer structure and more efficient compared to just using procedural programming.
C# Classes
To create a class in C#, we use the class keyword to declare a class.
class MyClass
{
private string name;
}
C# Objects
To create an object for the class we created we refer to the class name and use the new keyword to create a new reference to the class.
MyClass myObj = new MyClass();
C# Access Modifiers
Access modifiers are used to set the visibility of a class, field, method, and property.
-
public: accessible in any level
-
private: only accessible in the same class
-
protected: accessible in that class and any class derived.
C# Constructers & Destructors
You can set up Constructers in your class to set up default values for the fields inside of your class. A Destructor destroys instances of a class that are no longer needed.
class myClass
{
private string name;
private int age;
myClass()
{
name = "None";
age = 0;
}
myClass(string name, int age)
{
this.name = name;
this.age = age;
}
~myClass()
{
Console.WriteLine("Instance Destroyed");
}
}
You are able to set up many Constructers, but you can only have on Destructor.